Usage of very large numeric or string values are common in almost all encryption procedures. For this purposes we can't use the built-in data types in c#. That's where the Biginteger data type comes to our rescue. To use BigInteger functionality in c# we have to add the reference "vjslib" from the .NET tab.After adding the reference add the namespace "using java.math" in your project. Now we are ready to play with the Big Integer functionality in our C# application.
Below is an example of BigInteger multipication:
BigInteger xyz = new BigInteger("63478364346872346874682473687346873468");
BigInteger def = new BigInteger("36537845687346583465874365843756483756");
BigInteger abc = xyz.multiply(def); // abc contains the result.
Note that the BigInteger data type accepts input as "string" from which you can typecast to any type you need. Otherwise you will get the following error :
Error 3 Cannot implicitly convert type 'int' to 'java.math.BigInteger'
Enjoy!
Welcome to CodeGlobe. CodeGlobe provides free source code, tutorials and latest useful technology news.
Wednesday, January 28, 2009
Clearing all textbox and combobox in C#
The "foreach" statement repeats a group of embedded statements for each element in an array or an object collection .The "foreach" statement is used to iterate through the collection to get the information that you want, but can not be used to add or remove items from the source collection to avoid unpredictable side effects. For example we can loop through the controls with in a form or containers(eg: Panels,groupboxes etc).
in the below example we loop through all the controls in a groupbox and check whether it contains any textbox or combobox.If found then we clear its contents.
foreach (Control ctrl in groupBox2.Controls) //loop through controls in the groupbox
{
if (ctrl is TextBox)//checking whether the control is a textbox(Textbox is a class).
{
(ctrl as TextBox).Clear();
}
else
if(ctrl is ComboBox)//checking whether the control is a ComboBox(ComboBox is a class).
{
(ctrl as ComboBox).Text = "";
}
}
Simple isn't it.
in the below example we loop through all the controls in a groupbox and check whether it contains any textbox or combobox.If found then we clear its contents.
foreach (Control ctrl in groupBox2.Controls) //loop through controls in the groupbox
{
if (ctrl is TextBox)//checking whether the control is a textbox(Textbox is a class).
{
(ctrl as TextBox).Clear();
}
else
if(ctrl is ComboBox)//checking whether the control is a ComboBox(ComboBox is a class).
{
(ctrl as ComboBox).Text = "";
}
}
Simple isn't it.
Friday, January 23, 2009
Watermarking an image in C#.net
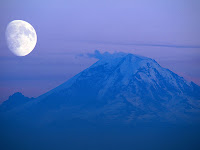
Then we select our watermark image.
Now open a new project and add the reference System.Drawing.Imaging;
Next type the following code. Each line in the following code is sufficiently commented.
Image image = System.Drawing.Image.FromFile(@"C:\Ascent.jpg");//This is the background image
Image logo = Image.FromFile(@"C:\SUNFLOWR.JPG"); //This is your watermark
Graphics g = System.Drawing.Graphics.FromImage(image); //Create graphics object of the background image //So that you can draw your logo on it
Bitmap TransparentLogo = new Bitmap(logo.Width, logo.Height); //Create a blank bitmap object //to which we //draw our transparent logo
Graphics TGraphics = Graphics.FromImage(TransparentLogo);//Create a graphics object so that //we can draw //on the blank bitmap image object
ColorMatrix ColorMatrix = new ColorMatrix(); //An image is represenred as a 5X4 matrix(i.e 4 //columns and 5 //rows)
ColorMatrix.Matrix33 = 0.25F;//the 3rd element of the 4th row represents the transparency
ImageAttributes ImgAttributes = new ImageAttributes();//an ImageAttributes object is used to set all //the alpha //values.This is done by initializing a color matrix and setting the alpha scaling value in the matrix.The address of //the color matrix is passed to the SetColorMatrix method of the //ImageAttributes object, and the //ImageAttributes object is passed to the DrawImage method of the Graphics object.
ImgAttributes.SetColorMatrix(ColorMatrix, ColorMatrixFlag.Default, ColorAdjustType.Bitmap); TGraphics.DrawImage(logo, new Rectangle(0, 0, TransparentLogo.Width, TransparentLogo.Height), 0, 0, TransparentLogo.Width, TransparentLogo.Height , GraphicsUnit.Pixel, ImgAttributes);
TGraphics.Dispose();
g.DrawImage(TransparentLogo, image.Width / 2, 30);
image.Save(@"C:\Test.jpeg", ImageFormat.Jpeg);
This is how the resulting image will look like.
kerala webdevelopers-webhosting providers
Subscribe to:
Posts (Atom)