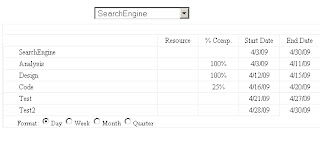
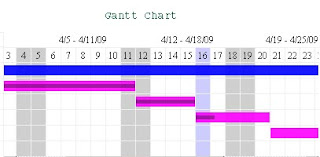
Gantt Chart in asp.net
A Gantt chart(wiki link) is a type of bar chart that illustrates a project schedule. Gantt charts illustrate the starting and finishing dates of terminal elements and summary elements of a project. Terminal elements and summary elements comprise the work breakdown structure of the project. Some Gantt charts also show the dependency (i.e, precedence network) relationships between activities.
We here use jsgantt(Its a free JavaScript library for creating Gantt chart) to create Gantt chart having following features .It’s implemented using JavaScript, HTML, CSS and thus very fast and flexible. Since it is pure HTML/JavaScript you can integrate this with any server side scripting language.
Basic Features
* Tasks & Collapsible Task Groups
* Multiple Dependencies
* Task Completion
* Task Color
* Milestones
* Resources
* No images needed
* Free [released under BSD license]
Advanced Features
* Dynamic Loading of Tasks
* Dynamic change of format (day/week/month)
* Load Gantt from XML file
Disadvantage
Currently only one Gantt chart is allowed per page.
You can download jsGantt from http://code.google.com/p/jsgantt/
There are many ways to integrate gantt chart programmatically, here we explain one of the method which using external data exchange between asp.net and JavaScript using XML file that are generated at run time using values from the SQL Server Database.
Sample xml file Structure that used by jsgantt. Is as follows
<project>
<task>
<pID>10</pID>
<pName>WCF Changes</pName>
<pStart></pStart>
<pEnd></pEnd>
<pColor>0000ff</pColor>
<pLink></pLink>
<pMile>0</pMile>
<pRes></pRes>
<pComp>0</pComp>
<pGroup>1</pGroup>
<pParent>0</pParent>
<pOpen>1</pOpen>
<pDepend />
</task>
<task>
<pID>20</pID>
<pName>Move to WCF from remoting</pName>
<pStart>
<pEnd>
<pColor>0000ff</pColor>
<pLink></pLink>
<pMile>0</pMile>
<pRes>Rich</pRes>
<pComp>10</pComp>
<pGroup>0</pGroup>
<pParent>10</pParent>
<pOpen>1</pOpen>
<pDepend></pDepend>
</task>
</project>
XML tags in our External XML file have following tags and values. Here we describe the purpose of each tag:
pID: (required) is a unique ID used to identify each row for parent functions and for setting dom id for hiding/showing
pName: (required) is the task Label
pStart: (required) the task start date, can enter empty date ('') for groups
pEnd: (required) the task end date, can enter empty date ('') for groups
pColor: (required) the html color for this task; e.g. '00ff00'
pLink: (optional) any http link navigated to when task bar is clicked.
pMile:(optional) represent a milestone
pRes: (optional) resource name
pComp: (required) completion percent
pGroup: (optional) indicates whether this is a group(parent) - 0=NOT Parent; 1=IS Parent
pParent: (required) identifies a parent pID, this causes this task to be a child of identified task
pOpen: can be initially set to close folder when chart is first drawn
pDepend: optional list of id's this task is dependent on ... line drawn from dependent to this item
pCaption: optional caption that will be added after task bar if Caption Type set to "Caption"
Creating Database Schema in Sql Server
Create database named Projects in SQL Server
Tables
project
Column Name --------------------------------Data Type
projectid-----------------------------------------Int(PK)
projectname-------------------------------------Varchar(50)
startdate-----------------------------------------Datetime
enddate------------------------------------------Datetime
tasks
Column Name---------------------------------- Data Type
taskid-------------------------------------------Int((PK)
projectid----------------------------------------Int(FK)
taskname---------------------------------------Varchar(50)
startdate----------------------------------------Datetime
enddate---------------------------------------- Datetime
percentagecompleted---------------------------Int
parenttask--------------------------------------Int
Sample data
project
projectid----------------------name------------------ startdate-------------- enddate
1---------------------------Search Engine-----------04/03/2009 -----------04/30/2009
Task
projectid name startdate enddate percentagecompleted parenttask
1--------------Analysis-----
2--------------Design-------
3--------------Code--------
4--------------Test ---------
5------------- Test2--------
*Tasks Date is in mm/dd/yyyy format
Using Gantt Chart in your ASP.NET Application
Create a website project in ASP.NET in Visual Studio.
Add an XML file named Tasks.xml to project
UnCompress Copy paste jsgantt directory you downloaded from jsGantt Website http://code.google.com/p/jsgantt/ to your project
Add dropdownlist control to Default.aspx to fill projects and to select name it cmbproject
Edit .aspx page’s HTML do following step
1. Include JSGantt CSS and Javascript
Add this just before the </head> tag
<link rel="stylesheet" type="text/css" href="jsgantt.css" />
<script language="javascript" src="jsgantt.js"></script>
2. Create a div element to hold the Gantt chart
In your html body
<div style="position:relative" class="gantt" id="GanttChartDIV"></div>
- Add This script to html body
<script>
var g = new JSGantt.GanttChart('g',document.getElementById('GanttChartDIV'), 'day');//Here intantiating chart control
g.setShowRes(1); // Show/Hide Responsible (0/1)
g.setShowDur(0); // Show/Hide Duration (0/1)
g.setShowComp(1); // Show/Hide % Complete(0/1)
//g.setCaptionType('Resource'); // Set to Show Caption (None,Caption,Resource,Duration,Complete)
if( g ) {
// You can also use the XML file parser
JSGantt.parseXML('Tasks.xml',g)//Read data from Tasks.xml
g.Draw();
g.DrawDependencies();
}
else
{
alert("not defined");
}
</script>
ASP.NET CODE
Default.aspx
<%@ Page Language="C#" AutoEventWireup="true" CodeFile="Default.aspx.cs" Inherits="_Default" %>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml" >
<head runat="server">
<title>Untitled Page</title>
<link rel="stylesheet" type="text/css" href="jsgantt/jsgantt.css"/>
<script language="javascript" src="jsgantt/jsgantt.js"></script>
</head>
<body>
<form id="form1" runat="server">
<div>
<table style="width: 384px">
<tr>
<td style="width: 185px">
</td>
<td style="width: 167px">
</td>
<td style="width: 65px">
</td>
</tr>
<tr>
<td style="width: 185px; height: 24px;">
</td>
<td style="width: 167px; height: 24px;">
<asp:DropDownList ID="cmbproject" runat="server" AutoPostBack="True" OnSelectedIndexChanged="cmbproject_SelectedIndexChanged"
Width="165px">
</asp:DropDownList></td>
<td style="width: 65px; height: 24px;">
</td>
</tr>
<tr>
<td style="width: 185px">
</td>
<td style="width: 167px">
</td>
<td style="width: 65px">
</td>
</tr>
</table>
</div>
<div style="position:relative" class="gantt" id="GanttChartDIV">
</div>
<script>
var g = new JSGantt.GanttChart('g',document.getElementById('GanttChartDIV'), 'day');
g.setShowRes(1); // Show/Hide Responsible (0/1)
g.setShowDur(0); // Show/Hide Duration (0/1)
g.setShowComp(1); // Show/Hide % Complete(0/1)
//g.setCaptionType('Resource'); // Set to Show Caption (None,Caption,Resource,Duration,Complete)
if( g ) {
// You can also use the XML file parser
JSGantt.parseXML('Tasks.xml',g)//Read data from Tasks.xml
g.Draw();
g.DrawDependencies();
}
else
{
alert("not defined");
}
</script>
</form>
</body>
</html>
Default.aspx.cs
using System;
using System.Data;
using System.Configuration;
using System.Web;
using System.Web.Security;
using System.Web.UI;
using System.Web.UI.WebControls;
using System.Web.UI.WebControls.WebParts;
using System.Web.UI.HtmlControls;
using System.Data.SqlClient;
using System.Xml;
public partial class _Default : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
if (!IsPostBack)
{
//Fill dropdownlist with all projects names
SqlConnection mcon = new SqlConnection("Data Source=CYBERMIND\\SQLEXPRESS;Database=Projects;Trusted_Connection=True"); //Cahnge Connection string of your SQL Server Here
mcon.Open();
cmbproject.Items.Clear();
SqlCommand GetProjectName = new SqlCommand("select projectid,name from project", mcon);
SqlDataReader ReadPrjName;
ReadPrjName = GetProjectName.ExecuteReader();
cmbproject.Items.Add("--------Select-----");
while (ReadPrjName.Read())
{
ListItem LIT = new ListItem();
LIT.Text = ReadPrjName.GetValue(1).ToString();//Add Project name as dropdownlists Text
LIT.Value = ReadPrjName.GetValue(0).ToString();//Add Projectid as dropdownlists Value
cmbproject.Items.Add(LIT);
}
ReadPrjName.Close();
mcon.Close();
}
}
protected void cmbproject_SelectedIndexChanged(object sender, EventArgs e)
{
string projectid = "";
SqlConnection mcon = new SqlConnection("Data Source=CYBERMIND\\SQLEXPRESS;Database=Projects;Trusted_Connection=True");
mcon.Open();
//Creating data of ou Task.xml programatically
XmlTextWriter xwriter = new XmlTextWriter(Server.MapPath("Tasks.xml"), null); //Creating a Xml Text writer
xwriter.Formatting = Formatting.Indented;// Setting indendation in xml file
xwriter.Indentation = 4;// Indentation space is 4 here
SqlCommand mmcmd = new SqlCommand("select projectid,name,convert(varchar(10),startdate,101),convert(varchar(10),enddate,101) from project where projectid =" + cmbproject.SelectedItem.Value + "", mcon);
SqlDataReader projectReader = mmcmd.ExecuteReader();
xwriter.WriteStartElement("project");
while (projectReader.Read())
{
projectid = projectReader.GetValue(0).ToString();
xwriter.WriteStartElement("task");
xwriter.WriteStartElement("pID"); // WriteStartElement writes a tag
xwriter.WriteString(projectReader.GetValue(0).ToString()); // WriteString writes strin to xml file
xwriter.WriteEndElement();// WriteStartElement writes a end tag
xwriter.WriteStartElement("pName");
xwriter.WriteString(projectReader.GetValue(1).ToString());
xwriter.WriteEndElement();
xwriter.WriteStartElement("pStart");
xwriter.WriteString(projectReader.GetValue(2).ToString());
xwriter.WriteEndElement();
xwriter.WriteStartElement("pEnd");
xwriter.WriteString(projectReader.GetValue(3).ToString());
xwriter.WriteEndElement();
xwriter.WriteStartElement("pParent");
xwriter.WriteString("0");
xwriter.WriteEndElement();
xwriter.WriteEndElement();
}
//End of Project Details Read and Write to XML
mcon.Close();
SqlConnection mcon2 = new SqlConnection("Data Source=CYBERMIND\\SQLEXPRESS;Database=Projects;Trusted_Connection=True");
mcon2.Open();
string str = "select taskid,taskname,convert(varchar(10),startdate,101),convert(varchar(10),enddate,101), percentagecompleted from Task where projectid =" + cmbproject.SelectedItem.Value + " ";
SqlCommand mcmd = new SqlCommand(str, mcon2);
SqlDataReader reader = mcmd.ExecuteReader();
//Start to read and write Tasks to Xml file
while (reader.Read())
{
xwriter.WriteStartElement("task");
xwriter.WriteStartElement("pID");
xwriter.WriteString(reader.GetValue(0).ToString());
xwriter.WriteEndElement();
xwriter.WriteStartElement("pName");
xwriter.WriteString(reader.GetValue(1).ToString());
xwriter.WriteEndElement();
xwriter.WriteStartElement("pStart");
xwriter.WriteString(reader.GetValue(2).ToString());
xwriter.WriteEndElement();
xwriter.WriteStartElement("pEnd");
xwriter.WriteString(reader.GetValue(3).ToString());
xwriter.WriteEndElement();
xwriter.WriteStartElement("pColor");// Sets chart task bar color to blue
xwriter.WriteString("ff00ff");
xwriter.WriteEndElement();
xwriter.WriteStartElement("pComp");
xwriter.WriteString(reader.GetValue(4).ToString());
xwriter.WriteEndElement();
xwriter.WriteStartElement("pParent");
xwriter.WriteString(projectid);
xwriter.WriteEndElement();
xwriter.WriteEndElement();
}
xwriter.WriteEndElement();
//Start to read and write Tasks to Xml file
xwriter.Flush();
xwriter.Close(); //Close XML File
mcon2.Close();
}
}
Muy buen post!!
ReplyDeletecomo puedo restaurar la Abrir la base de datos en sqlserver 2000 o solo es compatible con sqlexpress
Create a Database in Sql Server 2000 with the above given Table structure.Also fill the table with sample data given above.
ReplyDeleteAwesome post, I'm gonna study it and hopefully use it. Thanks for sharing and more power!
ReplyDelete-richard